For those studying C++ and in search of exemplary C++ exercises to deepen their understanding and elevate their C++ knowledge, we extend an invitation to explore and download the compilation of challenging C++ exercises with solutions shared below.
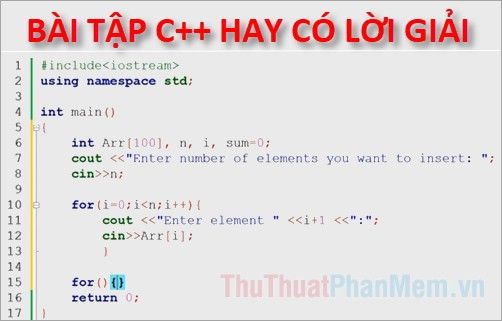
Presented here are fundamental C++ exercises with solutions. You can access additional basic and advanced C++ exercises via the link provided at the end of this article.
C++ Function Exercises
1. Find the greatest common divisor of two numbers, a and b.
#includeusing namespace std; int gcd(int, int); int gcd(int x, int y){ if (x < y){ x = x + y; y = x - y; x = x - y; } while (y != 0){ int m = x%y; x = y; y = m; } return x; } int main() { int a, b; cin >> a >> b; cout << gcd(a, b); return 0; }
2. Write a program using a function to check for a leap year.
#include#include int isLeapYear(int y) { return y % 4 == 0 && (y % 100 != 0 || y % 400 == 0);} void main() { int n; do { cin >> n; if (isLeapYear(n)) cout << n << ' is a leap year.\n'; else cout << n << ' is not a leap year.\n'; } while (n>1); }
C++ Control Statements Exercises
Input an integer, then output the hundreds digit of that number, or 0 if there isn't one.
Example:
Input | Output |
1234 |
2 |
#includeusing namespace std; int main() { int n, i; cin >> n; i = 0; if (n < 100) { cout << i << endl; } else { i = n / 100 % 10; cout << i << endl; } return 0; }
C++ String Exercises
1. Write a program to remove the character 'a' from a string.
#include#include #include void main() { int i,j; char x[80]; cout<<' Enter a string : '; gets(x); for (i=j=0;x[i]!=NULL;i++) if (x[i]!='a') { x[j]=x[i]; j++; } x[j]= NULL; cout<<' The string after removing 'a' is:'; puts(x); getch(); }
2. Write a program to extract the left substring of a string.
#include #include
#include void main()
{
clrscr();
char ten[25], *tentro; tentro=ten;
int i,sokytu;
cout
<<'
Enter a string of characters : '; gets(ten);
cout<<'
How many characters do you want to extract :'; cin>>sokytu;
for (i=0;i
C++ Array and Pointers Exercises
1. Find the smallest and largest numbers in an array.
#include
using namespace std;
int main()
{
int a[100];
int n = 0;
int x;
while (cin >> x) {
a[n] = x;
n++;
}
int maxval = a[0], minval = a[0];
for (int i = 1; i < n; i++) {
if (maxval < a[i])
maxval = a[i];
if (minval > a[i])
minval = a[i];
}
cout << minval << endl;
cout << maxval << endl;
return 0;
}
2. Write a program to input a real matrix of up to 20x20 and find the maximum value among the elements of the matrix.
#include#include void main() { float a[20][20],smax; int m,n,i,j,imax,jmax; clrscr(); puts('Enter the number of rows and columns of the matrix: '); scanf('%d%d',&m,&n); for (i=0;i
C++ Input Output Exercises
1. Input a character, then output its ASCII code.
Example:
Input | Output |
A |
65 |
#include
using namespace std;
int main()
{
char m;
cin >> m;
cout << int(m);
return 0;
}
2. Input a two-digit number, then output the sum of its digits to the screen.
Example:
Input | Output |
23 |
5 |
#include
using namespace std;
int main()
{
int a;
cin >> a;
cout << a%10 + a/10;
return 0;
}
C++ Loop Exercises
1. Input an integer n, output the sum of all odd numbers from 1 to n.
Example:
Input | Output |
3 |
4 |
#include
using namespace std;
int main()
{
int n , s = 0;
cin>>n;
for(int i=1; i<=n; i=i+2)
{
s = s+i;
}
cout << s;
return 0;
}
2. Input an integer n, output n!
Example:
Input | Output |
3 |
6 |
#include
using namespace std;
int main()
{
int n , factorial = 1;
cin >> n;
for (int i = 1; i <= n; i++) {
factorial = factorial*i;
}
cout << factorial << endl;
return 0;
}
C++ File Handling Exercises
Write a program to perform the following tasks:
- Input 10 real numbers into a text file named INPUT.
- Read the contents of file INPUT.
- Calculate the sum of squares of the numbers in file INPUT.
#include #include #include
void write()
{FILE *f = fopen('input','wt');
/*Write 10 real numbers into the text file*/
for (int i=1; i<=10;i++)
{ float a;
printf('\n Enter number %d: ',i); scanf('%f', &a);
fprintf(f,'%f ',a);
}
fclose(f);
}
void read()
{ int i;float a;
FILE *f = fopen('input','rt'); printf('\n Contents of the file are: \n\n'); do
{ fscanf(f,'%f',&a);
if (!feof(f))
printf('%.2f ',a);
} while (!feof(f)); fclose(f);
}
float tongbp()
{ int i;float tong = 0;
FILE *f = fopen('input','rt'); do
{ float a; fscanf(f,'%f',&a);
if (!feof(f)) tong+=a*a;
}while (!feof(f)); fclose(f);
return tong;
}
void main()
{ clrscr();
write();
read();
printf('\n Sum of squares is %.2f \n ',tongbp()); getch();
}
Download a collection of great C++ exercises with solutions here.
awesome-cpp-exercises-with-solutions.rar
So here we have compiled some excellent C++ exercises with solutions for you to download and review the code. Hope you will gain more valuable knowledge to enhance your skills in C++. Wishing you all the best for success!