If you've ventured into Internet Relay Chat (IRC), chances are you've encountered a bot at some point. Bots, akin to independent agents, seamlessly integrate into networks mimicking human interactions. They can be coded to respond to user commands or even engage in conversation. In this comprehensive guide, explore the various avenues available for creating an IRC bot and learn the intricacies of developing one from the ground up.
Guided Steps
Weighing Your Options
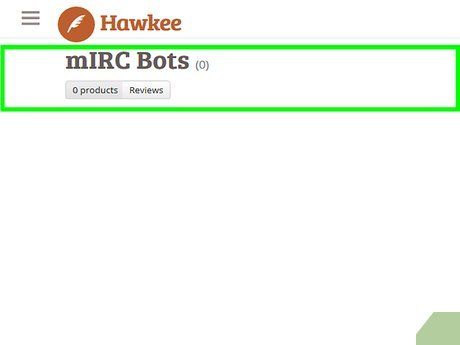
Exploring Installation of Client Scripts. Sometimes, simplicity is key, and you may prefer a solution that doesn't involve an independent program. In such instances, attaching a script to your IRC client proves beneficial. This approach is particularly popular with mIRC, boasting a robust scripting engine and a plethora of available scripts. It's the simplest option and highly recommended for those with limited or no programming experience. However, the subsequent instructions in this guide assume a basic understanding of programming principles.
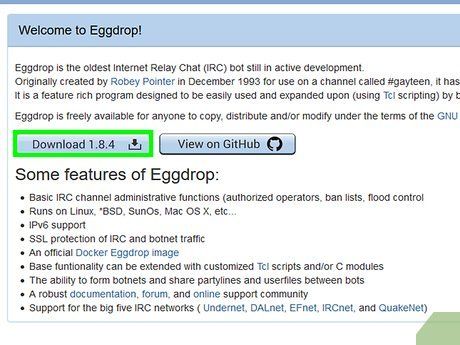
Exploring Existing Bot Frameworks. Numerous open-source and free applications are available to expedite the creation of your personalized bot. An exemplary instance is Eggdrop, hailed as the longest-standing IRC bot under continuous maintenance.
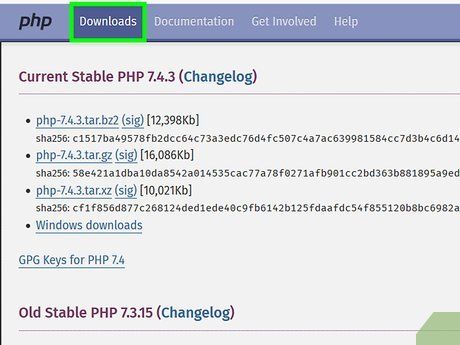
Delve into Bot Development. Tailored for proficient IRC enthusiasts and seasoned developers well-versed in programming languages, crafting a custom bot is an enticing prospect. Virtually any programming language with socket support suffices, with notable choices including Python, Lua, PHP, C, and Perl. Lack familiarity with these languages? Not an issue. Online resources offer examples in a myriad of languages. In this discourse, we'll illustrate using PHP. To utilize PHP, ensure PHP-CLI is installed on your system or server.
- PHP is obtainable from php.net
- PHP scripts can be executed from the command line. For additional guidance on PHP usage, refer to this PHP manual page.
Embarking on Bot Development
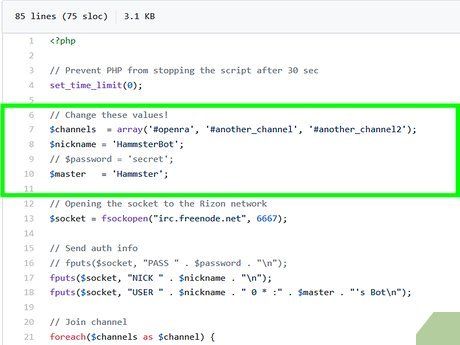
Acquire Connection Details. To establish a successful connection to the network, gather the following particulars:
-
Server: The domain name of the IRC server, such as
chat.freenode.net
- Port: Typically 6667, although verification from your IRC client or the network's website is prudent.
- Nickname: Your bot's designated moniker. Note that certain special characters are often prohibited (@#!~).
-
Ident: The ident field appears post-nickname in a WHOIS query, like this:
nickname!ident@hostname
- GECOS: Traditionally contains a user's real name or a brief bot description, but the content can vary.
- Channel: Typically prefaced with '#' on most networks, but this may vary.
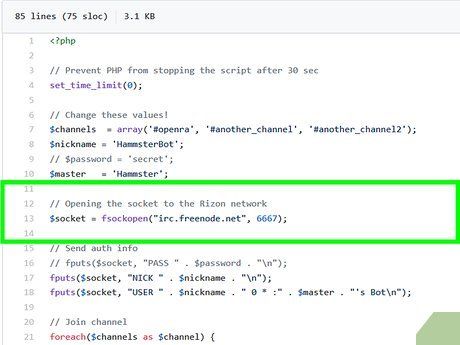
Configure Your Script. The fundamental approach involves defining variables corresponding to the aforementioned configuration aspects. Alternatively, storing them in a config file for parsing is feasible, but we'll focus on the essentials for now.
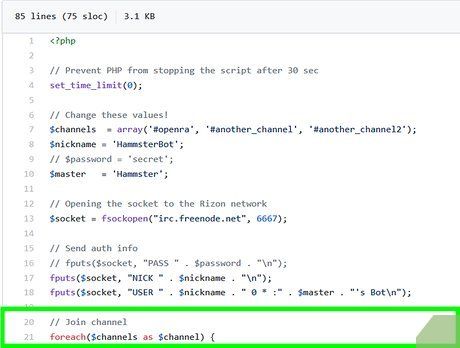
Establish Connection to the Network. Begin by initializing a socket connection to the server on the designated port. Implement error handling code to manage potential connection failures effectively. PHP offers convenient functions to streamline error management in such scenarios.
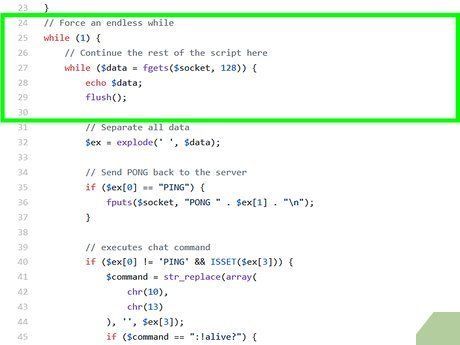
Enroll Your Bot. This involves furnishing your chosen nickname, ident, and GECOS details to the server, distinct from registering with NickServ. Simply transmit the NICK and USER commands to the server, adhering precisely to the format specified in RFC1459, the IRC protocol standard.
- Take note that the middle two parameters (in this case, * and 8) must be specified despite being disregarded by the server. These parameters are solely relevant between linked servers, not for a directly connecting client.
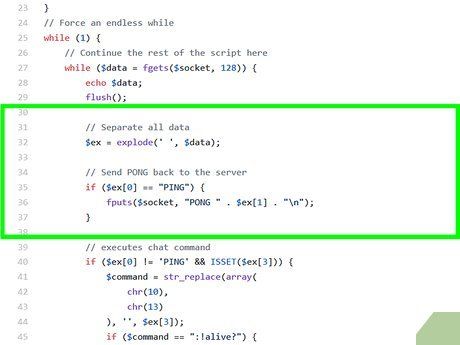
Continuously Retrieve Data from the Socket. Implement a loop to continually fetch data from the server via the socket. Failure to utilize a loop would result in immediate termination of the script, rendering the bot nonfunctional. Sustain the connection by retrieving and responding to any incoming data in the stream. Employ socket_read() to fetch available data, while simultaneously outputting raw data to the console for monitoring purposes.
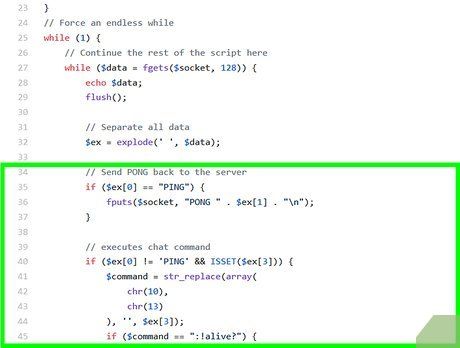
Implement a Ping Handler. This step is crucial to maintain connectivity. Promptly respond to pings from the server to prevent disconnection. Pings from the server typically follow this format:
PING :servername
. The server may specify any content following the ':'. It is imperative to echo back precisely what the server sent, substituting PONG for PING.
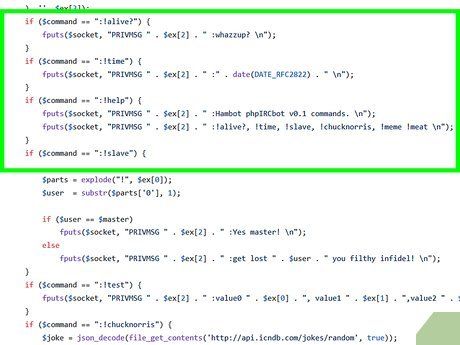
Join Your Channels. Now that your bot is connected and responsive to pings, it's time to facilitate interaction by joining channels. Without joining channels, your bot would be limited to responding only to private messages.
- To achieve this, monitor server status codes 376 or 422. Code 376 indicates the completion of the MOTD (message of the day), while 422 signifies the absence of an MOTD. The MOTD serves as an indicator for initiating channel joining.
- Issue a JOIN command, followed by one or more channel names separated by commas.
- The server transmits data conveniently delimited by spaces, allowing for easy parsing and reference using array indices.
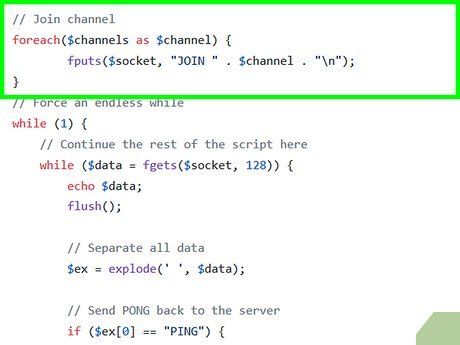
Engage with Channel Messages. Let's dive into the exciting realm of channel interactions. Now that your bot has joined the channel, it's primed for action. Let's create a sample command called @moo.
- Identify the message offset (applicable to both channels and private messages), consistently located in the same position.
- Handling commands with spaces involves reassembling the chunked data ($d), a topic beyond the scope of this discussion.
- When responding, distinguish between channels (e.g., #botters-test) and private messages. In the case of private messages, reply using the sender's nickname, not your own (otherwise, you'll be conversing with yourself, which is rather whimsical).
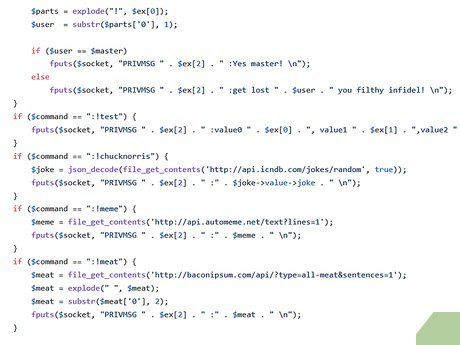
Enhance Your Bot's Functionality. Leveraging the aforementioned approach opens avenues for incorporating numerous additional features. The IRC network supports a plethora of other commands, including op management, kicking, banning, topic setting, and various other capabilities.
Insights
-
Emulate the '/me' Command by prefixing messages with:
- PRIVMSG #channel :\001ACTION text here\001.
- \001 denotes ASCII character 1, interpreted accordingly in a double-quoted PHP string. Alternatively, utilize
chr(1)
outside the string.
-
Introduce Colors into Messages by prefixing with '\003' (ASCII code 3), followed by a color code. For instance, 0 = white, 1 = black, 2 = blue, 3 = green, 4 = red [...]. Refer to mIRC's color chart for additional options.
-
Exercise Courtesy by obtaining consent from channel owners and IRC operators before deploying your bot. Not all networks and channels embrace bots, regardless of their conduct.